ToDo list is a list to manage your tasks, projects, and team work. And this article is creating a ToDo list using HTML, CSS, and JavaScript. And source code, you can get from (Tilak Jain): GitHub
HTML/CSS/JS Contents:
Add a link on the head of index.html to make some style
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.1/css/all.min.css" integrity="sha512-MV7K8+y+gLIBoVD59lQIYicR65iaqukzvf/nwasF0nqhPay5w/9lJmVM2hMDcnK1OnMGCdVK+iQrJ7lzPJQd1w==" crossorigin="anonymous" referrerpolicy="no-referrer" />
1 – Create index.html
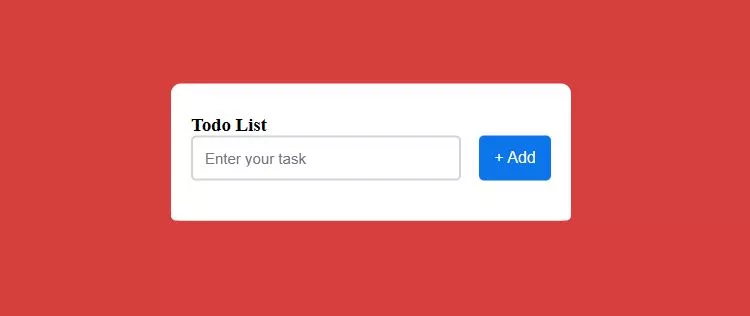
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width">
<link rel="stylesheet" href="./style.css">
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/6.2.1/css/all.min.css" integrity="sha512-MV7K8+y+gLIBoVD59lQIYicR65iaqukzvf/nwasF0nqhPay5w/9lJmVM2hMDcnK1OnMGCdVK+iQrJ7lzPJQd1w==" crossorigin="anonymous" referrerpolicy="no-referrer" />
<title>JavaScript - Todo List</title>
</head>
<body>
<div class="container">
<div id="newtask">
<h3>Todo List</h3>
<input type="text" placeholder="Enter your task">
<button id="push">+ Add</button>
</div>
<div id="tasks"></div>
</div>
<script src="./script.js"></script>
</body>
</html>
2 – Create CSS / StyleSheet (style.css)
*,
*:before,
*:after{
padding: 0;
margin: 0;
box-sizing: border-box;
}
body{
height: 100vh;
background: #d73f3f;
}
.container{
width: 40%;
top: 50%;
left: 50%;
background: white;
border-radius: 10px;
min-width: 300px;
position: absolute;
min-height: 100px;
transform: translate(-50%,-50%);
}
#newtask{
position: relative;
padding: 30px 20px;
}
#newtask input{
width: 75%;
height: 45px;
padding: 12px;
color: #111111;
font-weight: 500;
position: relative;
border-radius: 5px;
font-family: 'Poppins',sans-serif;
font-size: 15px;
border: 2px solid #d1d3d4;
}
#newtask input:focus{
outline: none;
border-color: #0d75ec;
}
#newtask button{
position: relative;
float: right;
font-weight: 500;
font-size: 16px;
background-color: #0d75ec;
border: none;
color: #ffffff;
cursor: pointer;
outline: none;
width: 20%;
height: 45px;
border-radius: 5px;
font-family: 'Poppins',sans-serif;
}
#tasks{
border-radius: 10px;
width: 100%;
position: relative;
background-color: #ffffff;
padding: 5px 20px;
}
.task{
border-radius: 5px;
align-items: center;
justify-content: space-between;
border: 1px solid #939697;
cursor: pointer;
background-color: #c5e1e6;
height: 50px;
margin-bottom: 8px;
padding: 5px 10px;
display: flex;
}
.task span{
font-family: 'Poppins',sans-serif;
font-size: 15px;
font-weight: 400;
}
.task button{
background-color: #0a2ea4;
color: #ffffff;
border: none;
cursor: pointer;
outline: none;
height: 100%;
width: 40px;
border-radius: 5px;
}
3 – Create JavaScript (script.js)
document.querySelector('#push').onclick = function(){
if(document.querySelector('#newtask input').value.length == 0){
alert("Please Enter a Task")
}
else{
document.querySelector('#tasks').innerHTML += `
<div class="task">
<span id="taskname">
${document.querySelector('#newtask input').value}
</span>
<button class="delete">
<i class="far fa-trash-alt"></i>
</button>
</div>
`;
var current_tasks = document.querySelectorAll(".delete");
for(var i=0; i<current_tasks.length; i++){
current_tasks[i].onclick = function(){
this.parentNode.remove();
}
}
}
}
Final Result:
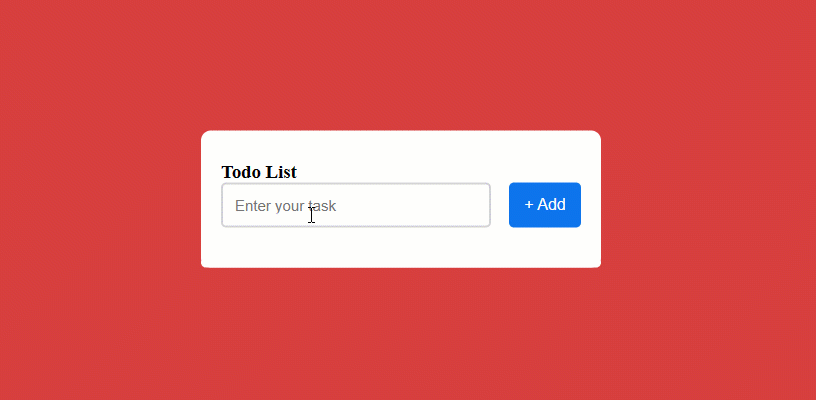
Sweet Navigation Menu using HTML, CSS & JavaScript
Hi everyone, this article is so sweet for you. I have new HTML, CSS, and JavaScript examples for creating a lovely navigation menu. it’s a credit to YT (Online Tutorials) they have a lot of beautiful tutorials about HTML, CSS, and Javascript.
In index.html, add JavaScript code at the bottom of the body code inside the <body> tag for creating an event when you click on a menu.
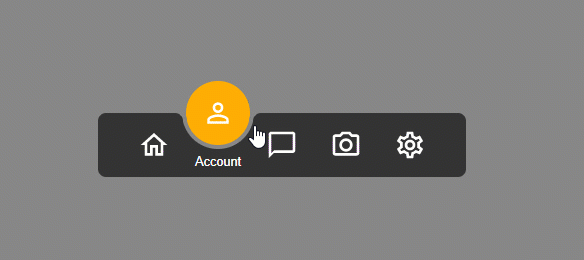
Related Articles
- Automatically Setting the Current Date and Time in an HTML Form
- How to Create a Spinner with Loading Data Percentage in DataTables
- Convert Numbers to Khmer Number System Words (HTML+JAVASCRIPT)
- Converting Numbers to Words in JavaScript
- Create Login Form Using HTML, CSS, JavaScript
- JavaScript – Create Notes App