You can create an app for counting numbers using JavaScript to help users count items or products or something.
And this article is creating a Counter app using HTML, CSS, and JavaScript. And source code, you can get from (Tilak Jain): GitHub
HTML/CSS/JavaScript Contents:
1. Create an HTML file (index.html)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Counter App</title>
<link rel="stylesheet" href="./style.css">
</head>
<body>
<h1>Counter App</h1>
<p class="counter-preview">0</p>
<div class="buttons" id="allBtns">
<button class="decrement" id="decrement">
Decrement
</button>
<button class="reset" id="reset">Reset</button>
<button class="increment" id="increment">Increment</button>
</div>
<script src="./script.js"></script>
</body>
</html>
2. Create CSS StyleSheet (style.css)
*{
box-sizing: border-box;
margin: 0;
}
body {
font-family: 'Open Sans', sans-serif;
font-size: 16px;
color: #333;
width: 400px;
margin: 0 auto;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
min-height: 90vh;
background: #A8DCF0;
}
h1 {
text-transform: uppercase;
}
.counter-preview {
font-size: 8rem;
font-weight: bold;
color: #333;
}
button {
color: #fff;
border: none;
padding: 10px 20px;
border-radius: 5px;
font-size: 18px;
cursor: pointer;
margin-top: 20px;
margin-left: 1rem;
}
.decrement {
background-color: #4caf50;
}
.reset {
background-color: #f44336;
}
.increment {
background-color: #2196f3;
}
A result of HTML & CSS, after your coding
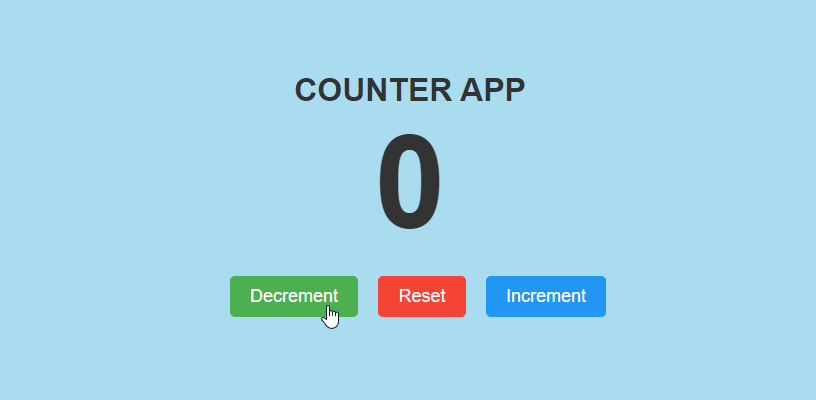
3. Create JavaScript (script.js)
const display = document.querySelector('.counter-preview');
const allBtns = document.querySelector('#allBtns');
allBtns.addEventListener('click', counter);
let value = 0;
function counter(e) {
const btn = e.target.id;
if (btn === 'increment') {
value += 1;
} else if (btn === 'decrement') {
value -= 1;
} else {
value = 0;
}
display.textContent = value;
}
Final Result:
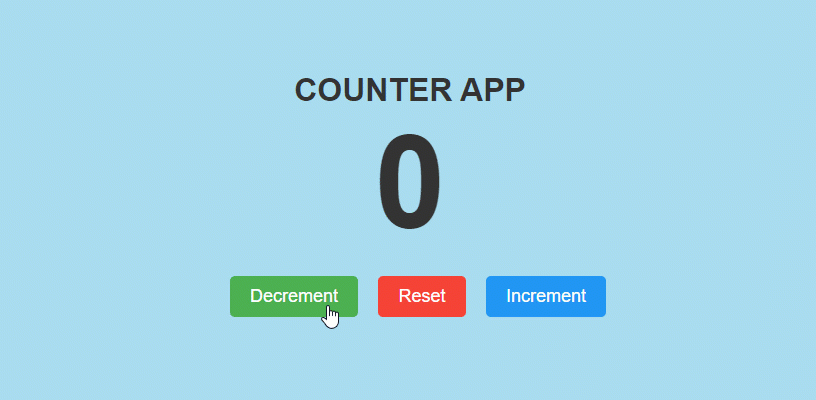
JavaScript – Digital Clock with Date
A digital clock is a type of clock that displays the time digitally (i.e. in numerals or other symbols), as opposed to an analog clock.
And this article is creating a Digital Clock using HTML, CSS, and JavaScript.
JavaScript – How to create Stopwatch
A Stopwatch is a timepiece designed to measure the amount of time that elapses between its activation and deactivation. A mechanical stopwatch.
And this article is creating a Stopwatch using HTML, CSS, and JavaScript.