A Stopwatch is a timepiece designed to measure the amount of time that elapses between its activation and deactivation. A mechanical stopwatch.
And this article is creating a Stopwatch using HTML, CSS, and JavaScript. And source code, you can get from (Tilak Jain): GitHub
HTML Contents
So you can follow the following code to create Stopwatch using JavaScript
1. Create an HTML file (index.html)
Add 3 buttons to create an event click like Start, Stop, and Reset
<!DOCTYPE html>
<html lang="en" xmlns="http://www.w3.org/1999/xhtml">
<head>
<meta charset="utf-8" />
<title></title>
<link rel="stylesheet" href="./style.css">
<script src="./script.js"></script>
</head>
<body>
<div class="wrapper">
<h2>Stopwatch</h2>
<p><span id="seconds">00</span>:<span id="tens">00</span></p>
<button id="button-start">Start</button>
<button id="button-stop">Stop</button>
<button id="button-reset">Reset</button>
</div>
</body>
</html>
2. Create a StyleSheet file (style.css)
The styleSheet is makeup and colors your page and button style
body {
background: #25282a;
font-family: sans-serif;
display: grid ;
height: 100%;
}
.wrapper {
width: 400px;
height: 100%;
border-radius: 5px;
border: solid 1px #fff;
margin: 30px auto;
color: #fff;
text-align: center;
}
h2,
h3 {
font-family: "Roboto", sans-serif;
font-weight: 500;
font-size: 2.6em;
text-transform: uppercase;
}
#seconds,
#tens {
font-size: 2em;
}
#button-start {
-moz-border-radius: 5px;
-webkit-border-radius: 5px;
border-radius: 5px;
-khtml-border-radius: 5px;
background: #41cd38;
color: #25282a;
border: solid 1px #fff;
text-decoration: none;
cursor: pointer;
font-size: 1.2em;
padding: 18px 10px;
width: 180px;
margin: 10px;
outline: none;
}
#button-stop {
-moz-border-radius: 5px;
-webkit-border-radius: 5px;
border-radius: 5px;
-khtml-border-radius: 5px;
background: #fb5b5b;
color: #25282a;
border: solid 1px #fff;
text-decoration: none;
cursor: pointer;
font-size: 1.2em;
padding: 18px 10px;
width: 180px;
margin: 10px;
outline: none;
}
button {
-moz-border-radius: 5px;
-webkit-border-radius: 5px;
border-radius: 5px;
-khtml-border-radius: 5px;
background: #a7dbf1;
color: #25282a;
border: solid 1px #fff;
text-decoration: none;
cursor: pointer;
font-size: 1.2em;
padding: 18px 10px;
width: 180px;
margin: 10px;
outline: none;
}
button:hover,#button-start:hover,#button-stop:hover {
-webkit-transition: all 0.5s ease-in-out;
-moz-transition: all 0.5s ease-in-out;
transition: all 0.5s ease-in-out;
background: #25282a;
border: solid 1px #a7dbf1;
color: #a7dbf1;
}
Result from HTML/CSS, after your coding with index.html and style.css
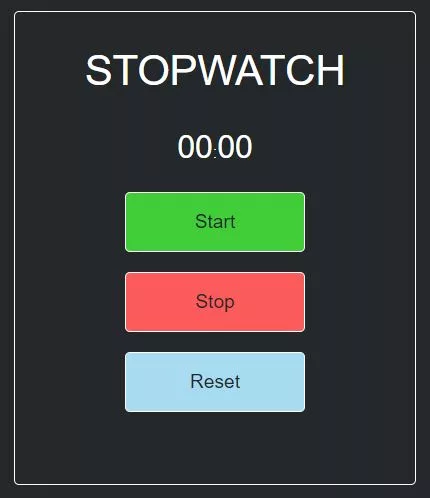
3. Create JavaScript (script.js)
The JavaScript creates an events click
The start button starts the stopwatch time
The stop button stops the stopwatch time
The reset button is reset to zero of the stopwatch time
window.onload = function () {
var seconds = 00;
var tens = 00;
var appendTens = document.getElementById("tens");
var appendSeconds = document.getElementById("seconds");
var buttonStart = document.getElementById("button-start");
var buttonStop = document.getElementById("button-stop");
var buttonReset = document.getElementById("button-reset");
var Interval;
buttonStart.onclick = function () {
clearInterval(Interval);
Interval = setInterval(startTimer, 10);
};
buttonStop.onclick = function () {
clearInterval(Interval);
};
buttonReset.onclick = function () {
clearInterval(Interval);
tens = "00";
seconds = "00";
appendTens.innerHTML = tens;
appendSeconds.innerHTML = seconds;
};
function startTimer() {
tens++;
if (tens <= 9) {
appendTens.innerHTML = "0" + tens;
}
if (tens > 9) {
appendTens.innerHTML = tens;
}
if (tens > 99) {
console.log("seconds");
seconds++;
appendSeconds.innerHTML = "0" + seconds;
tens = 0;
appendTens.innerHTML = "0" + 0;
}
if (seconds > 9) {
appendSeconds.innerHTML = seconds;
}
}
};
Final Result:
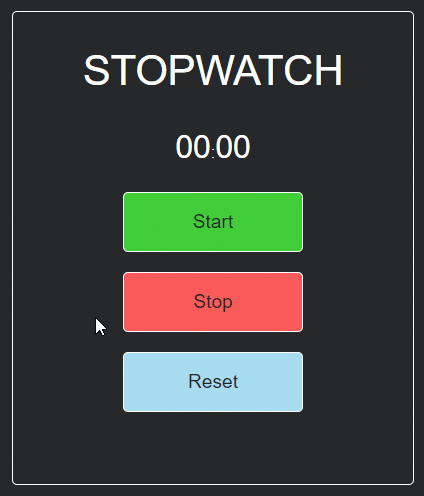
JavaScript – ToDo List
A ToDo list is a list to manage your tasks, projects, and teamwork. And this article is creating a ToDo list using HTML, CSS, and JavaScript. And source code.
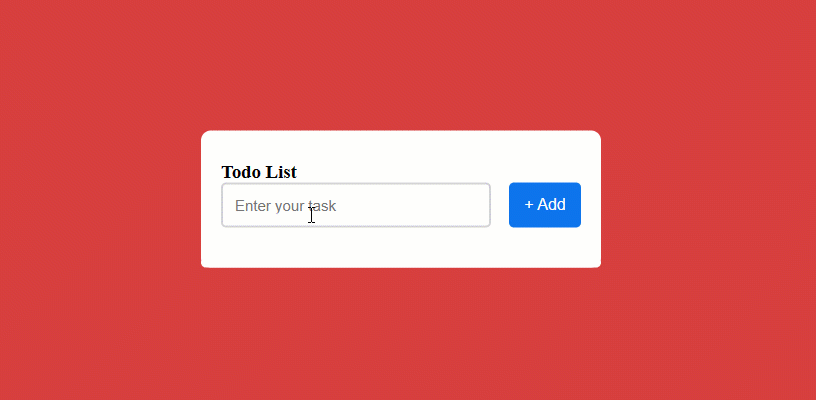