Kestrel is a cross-platform web server for ASP.NET Core. It is the default web server included in ASP.NET Core project templates and is designed to be fast and lightweight. Here’s a brief overview of Kestrel and its role in an ASP.NET Core application:
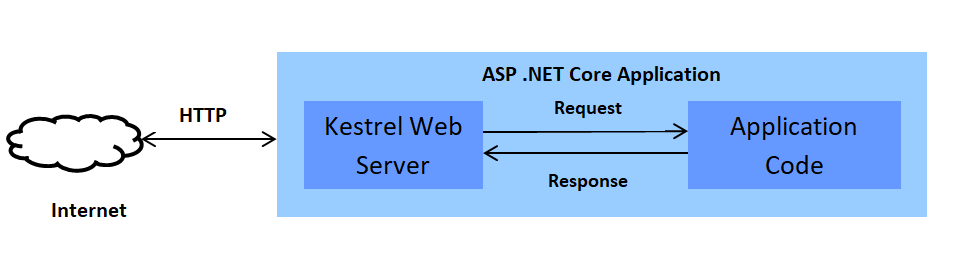
Key Features of Kestrel:
- Cross-Platform: Kestrel runs on Windows, macOS, and Linux, making it versatile for various deployment environments.
- High Performance: Kestrel is designed for high performance and low overhead, suitable for serving high-traffic applications.
- Lightweight: It is a lightweight web server, which makes it ideal for microservices and containerized applications.
- Integration: Kestrel can be used directly as the web server for ASP.NET Core applications or as an edge server in conjunction with a reverse proxy like IIS, Nginx, or Apache.
Typical Usage:
In a typical ASP.NET Core application, Kestrel is used as the internal web server. You can configure Kestrel directly in your application or rely on defaults provided by the ASP.NET Core framework.
Sample Diagram
Here’s a textual representation of how you might set up your diagram:
[Internet]
|
v
[Reverse Proxy (IIS/Nginx/Apache)]
|
v
[Kestrel]
|
v
[ASP.NET Core Application]
Example Configuration:
Here’s an example of how you might configure Kestrel in your Startup.cs
or Program.cs
file:
In Program.cs
(for ASP.NET Core 6.0 and later):
var builder = WebApplication.CreateBuilder(args);
builder.WebHost.UseKestrel(options =>
{
options.Limits.MaxRequestBodySize = 52428800; // 50 MB
options.Limits.KeepAliveTimeout = TimeSpan.FromMinutes(2);
options.AddServerHeader = false;
});
builder.Services.AddControllersWithViews();
var app = builder.Build();
app.UseRouting();
app.UseAuthorization();
app.MapDefaultControllerRoute();
app.Run();
In Startup.cs
(for earlier versions of ASP.NET Core):
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.Configure<KestrelServerOptions>(options =>
{
options.Limits.MaxRequestBodySize = 52428800; // 50 MB
options.Limits.KeepAliveTimeout = TimeSpan.FromMinutes(2);
});
services.AddControllersWithViews();
}
public void Configure(IApplicationBuilder app, IWebHostEnvironment env)
{
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
else
{
app.UseExceptionHandler("/Home/Error");
app.UseHsts();
}
app.UseHttpsRedirection();
app.UseStaticFiles();
app.UseRouting();
app.UseAuthorization();
app.UseEndpoints(endpoints =>
{
endpoints.MapControllerRoute(
name: "default",
pattern: "{controller=Home}/{action=Index}/{id?}");
});
}
}
For Kestrel, the configuration in Startup.cs
should be sufficient, but you can also configure it in appsettings.json
:
{
"Kestrel": {
"Limits": {
"MaxRequestBodySize": 52428800 // 50 MB
}
}
}
Integration with Reverse Proxies:
Kestrel is often used in combination with a reverse proxy, such as IIS, Nginx, or Apache. This setup can provide additional security, load balancing, and other features not provided by Kestrel alone.
Example of using Kestrel with IIS:
- Kestrel: Handles the HTTP requests.
- IIS: Acts as a reverse proxy, forwarding requests to Kestrel.
Why Use Kestrel?
- Performance: It’s designed for high performance and can handle high loads efficiently.
- Flexibility: It allows you to run your application in various environments and can be easily configured.
- Simplicity: Being lightweight, it’s suitable for microservices and containerized applications.
By default, when you create a new ASP.NET Core project, it is set up to use Kestrel. You can customize its settings as shown in the examples above to suit your application’s needs.