In the Android app, you see many apps that have popular social media applications sign up and sign in for using their app. Popular Social media have Facebook, Google accounts, Twitter …
For this example, I create a simple login project interacting with Google sign-in in Android Studio.
Android Contents:
1. Configure a Project interacting with Google Sign-in
First, you can get the project layout on GitHub and download here , it has a background image and icons. but you can design them by yourself.
Second, create a developer account at Google Identity and find Configure a Google API Console project and click on Configure a project. Popup screen select or create a project, click on + Create a new project and enter your project name, Next
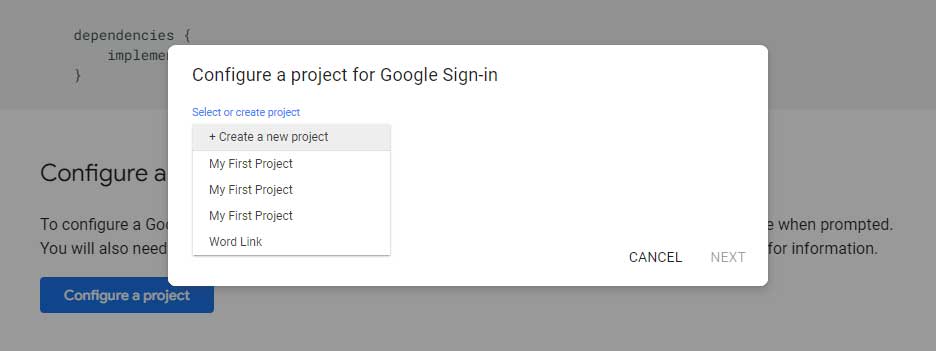
On Configure your OAuth client screen, enter your product name, and Next.
next screen, Select Android in the dropdown list.
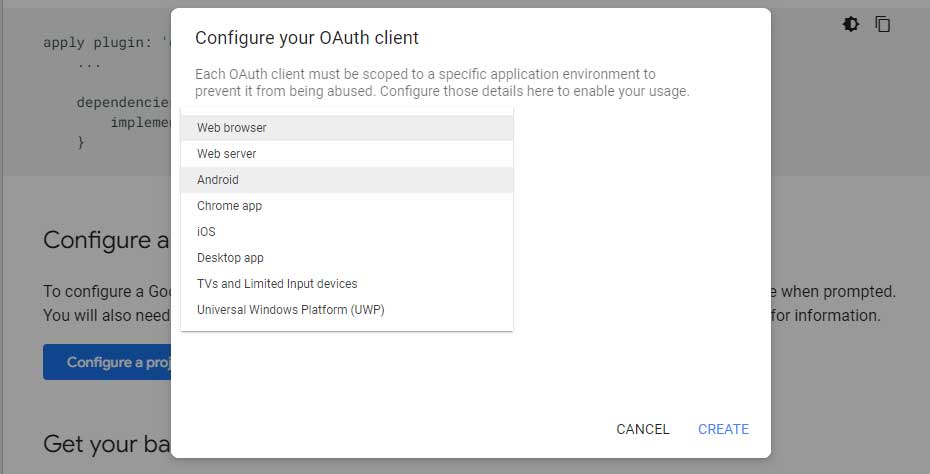
After selected, for getting the package name and SHA-1 signing certificate go to Android Studio, and create a new android project
For this example:
Project name: Simple User Login
Package name: com.eangkor.simpleuserlogin
Language: Java
On the top-right side of the Android project screen, click on Gradle and go to SigningReport
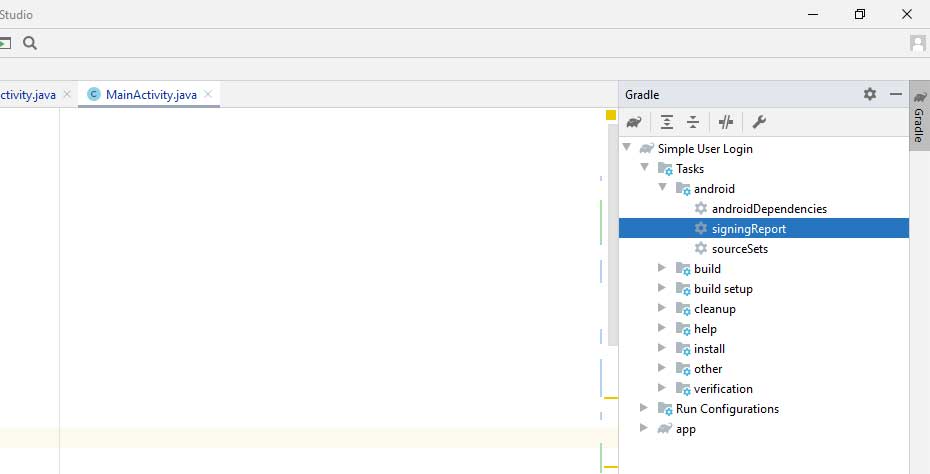
Double-Click on it or right-click and choose Run [signingReport], you will get an SHA-1 signing certificate at the bottom side of the screen, copy SHA1
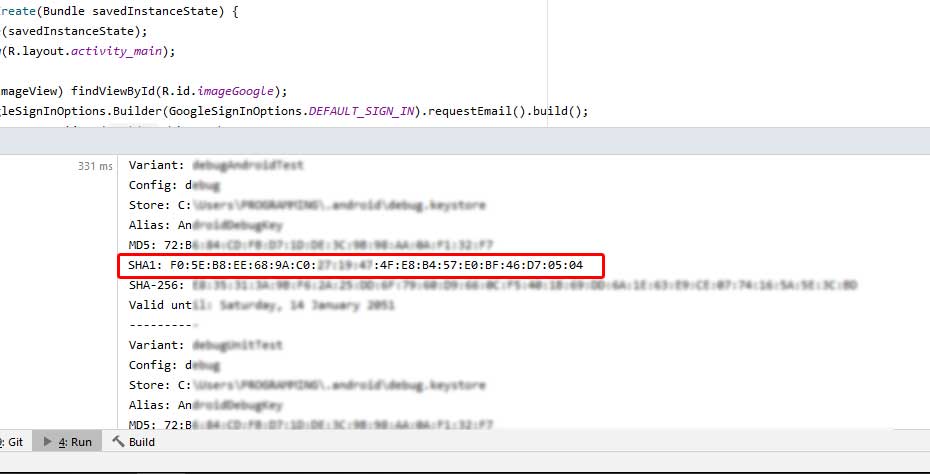
and paste it with the Package name into Package name box and SHA-1 signing certificate box of Google, and click on CREATE
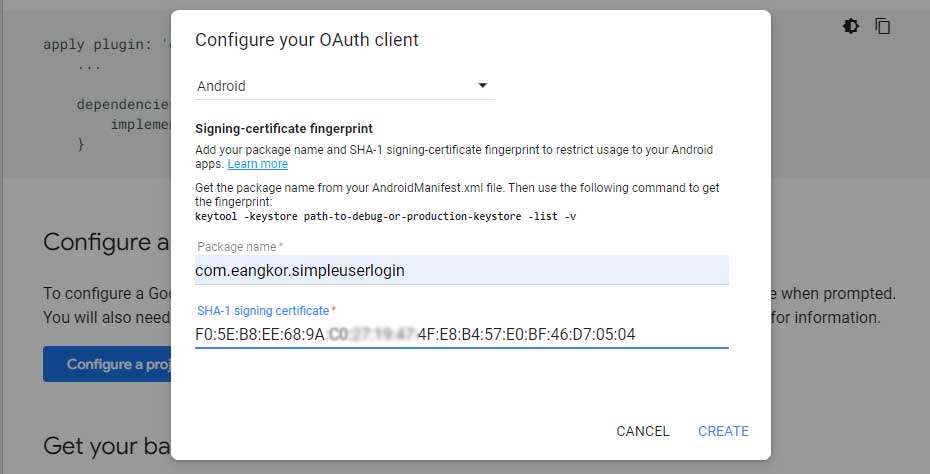
2. Start your coding
First, add the following statement line at dependency in build.gradle (Module) and click on Sync now
dependencies {
implementation 'com.google.android.gms:play-services-auth:20.2.0'
}
in AndroidMainifest.xml
Add Internet permission in AndroidMainifest
<uses-permission android:name="android.permission.INTERNET"/>
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.eangkor.simpleuserlogin">
<uses-permission android:name="android.permission.INTERNET"/>
<application
android:allowBackup="true"
android:icon="@mipmap/ic_launcher"
android:label="@string/app_name"
android:roundIcon="@mipmap/ic_launcher_round"
android:supportsRtl="true"
android:theme="@style/Theme.SimpleUserLogin">
<activity android:name=".WelcomeActivity" />
<activity
android:name=".MainActivity"
android:screenOrientation="portrait">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
In activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:background="@drawable/background"
tools:context=".MainActivity">
<EditText
android:id="@+id/editTextUsername"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:layout_marginTop="10dp"
android:background="#30ffffff"
android:drawableRight="@drawable/ic_action_user"
android:drawablePadding="10dp"
android:hint="Username"
android:inputType="textPersonName"
android:padding="20dp"
android:textColor="@color/white"
android:textColorHint="@color/white"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="1.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/imageView" />
<EditText
android:id="@+id/editTextPassword"
android:layout_width="0dp"
android:layout_height="wrap_content"
android:layout_margin="10dp"
android:background="#30ffffff"
android:drawableRight="@drawable/ic_action_info"
android:drawablePadding="10dp"
android:ems="10"
android:hint="Password"
android:inputType="textPassword"
android:padding="20dp"
android:textColor="@color/white"
android:textColorHint="@color/white"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="1.0"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextUsername" />
<Button
android:id="@+id/btnLogin"
android:layout_width="118dp"
android:layout_height="59dp"
android:layout_marginTop="20dp"
android:text="LOGIN"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.498"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/editTextPassword" />
<ImageView
android:id="@+id/imageView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="30dp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent"
app:srcCompat="@drawable/user_icon" />
<TextView
android:id="@+id/tvForget"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Forgot password?"
android:textColor="#FFFFFF"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.335"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/btnLogin" />
<TextView
android:id="@+id/tvSignup"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Sign Up"
android:textColor="#FFFFFF"
android:textSize="18sp"
android:textStyle="bold"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.106"
app:layout_constraintStart_toEndOf="@+id/tvForget"
app:layout_constraintTop_toBottomOf="@+id/btnLogin" />
<TextView
android:id="@+id/textView2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:text="Or sign in with"
android:textColor="#3F51B5"
app:layout_constraintBottom_toTopOf="@+id/imageGoogle"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
<ImageView
android:id="@+id/imageView2"
android:layout_width="40dp"
android:layout_height="40dp"
android:layout_marginEnd="20dp"
android:layout_marginBottom="32dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toStartOf="@+id/imageGoogle"
app:srcCompat="@drawable/fb" />
<ImageView
android:id="@+id/imageGoogle"
android:layout_width="40dp"
android:layout_height="40dp"
android:layout_marginBottom="32dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:srcCompat="@drawable/google" />
<ImageView
android:id="@+id/imageView4"
android:layout_width="40dp"
android:layout_height="40dp"
android:layout_marginStart="20dp"
android:layout_marginBottom="32dp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintStart_toEndOf="@+id/imageGoogle"
app:srcCompat="@drawable/twitter" />
</androidx.constraintlayout.widget.ConstraintLayout>
In welcome_activity.xml
<?xml version="1.0" encoding="utf-8"?>
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".WelcomeActivity">
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="20dp"
android:text="Welcome to my APP"
android:textSize="18sp"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toTopOf="parent" />
<TextView
android:id="@+id/textView3"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="www.ifixproblem.com"
android:textSize="18sp"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintHorizontal_bias="0.504"
app:layout_constraintStart_toStartOf="parent"
app:layout_constraintTop_toBottomOf="@+id/textView"
app:layout_constraintVertical_bias="0.027" />
<Button
android:id="@+id/btnlogout"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginBottom="20dp"
android:text="Logout"
app:layout_constraintBottom_toBottomOf="parent"
app:layout_constraintEnd_toEndOf="parent"
app:layout_constraintStart_toStartOf="parent" />
</androidx.constraintlayout.widget.ConstraintLayout>
MainActivity.java
package com.eangkor.simpleuserlogin;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.Toast;
import com.google.android.gms.auth.api.signin.GoogleSignIn;
import com.google.android.gms.auth.api.signin.GoogleSignInAccount;
import com.google.android.gms.auth.api.signin.GoogleSignInClient;
import com.google.android.gms.auth.api.signin.GoogleSignInOptions;
import com.google.android.gms.common.api.ApiException;
import com.google.android.gms.tasks.Task;
public class MainActivity extends AppCompatActivity {
GoogleSignInOptions gso;
GoogleSignInClient gsc;
ImageView imggoogle;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
imggoogle = (ImageView) findViewById(R.id.imageGoogle);
gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN).requestEmail().build();
gsc = GoogleSignIn.getClient(this,gso);
GoogleSignInAccount acct = GoogleSignIn.getLastSignedInAccount(this);
if(acct!=null){
navigateToSecondActivity();
}
imggoogle.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
signIn();
}
});
}
void signIn(){
Intent signInIntent = gsc.getSignInIntent();
startActivityForResult(signInIntent,1000);
}
@Override
protected void onActivityResult(int requestCode, int resultCode,Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if(requestCode == 1000){
Task<GoogleSignInAccount> task = GoogleSignIn.getSignedInAccountFromIntent(data);
try {
task.getResult(ApiException.class);
navigateToSecondActivity();
} catch (ApiException e) {
Toast.makeText(getApplicationContext(), "Something went wrong!", Toast.LENGTH_SHORT).show();
}
}
}
void navigateToSecondActivity(){
finish();
Intent intent = new Intent(MainActivity.this,WelcomeActivity.class);
startActivity(intent);
}
}
WelcomeActivity.java
package com.eangkor.simpleuserlogin;
import androidx.appcompat.app.AppCompatActivity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.Toast;
import com.google.android.gms.auth.api.Auth;
import com.google.android.gms.auth.api.signin.GoogleSignInClient;
import com.google.android.gms.auth.api.signin.GoogleSignInOptions;
import com.google.android.gms.common.api.GoogleApiClient;
import com.google.android.gms.common.api.ResultCallback;
import com.google.android.gms.common.api.Status;
public class WelcomeActivity extends AppCompatActivity {
Button btn_logout;
private GoogleApiClient gac;
private GoogleSignInClient gsc;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_welcome);
btn_logout=(Button)findViewById(R.id.btnlogout);
btn_logout.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Auth.GoogleSignInApi.signOut(gac).setResultCallback(
new ResultCallback<Status>() {
@Override
public void onResult(Status status) {
// ...
Toast.makeText(getApplicationContext(),"Logged Out",Toast.LENGTH_SHORT).show();
Intent i=new Intent(getApplicationContext(),MainActivity.class);
startActivity(i);
}
});
}
});
}
@Override
protected void onStart() {
GoogleSignInOptions gso = new GoogleSignInOptions.Builder(GoogleSignInOptions.DEFAULT_SIGN_IN)
.requestEmail()
.build();
gac = new GoogleApiClient.Builder(this)
.addApi(Auth.GOOGLE_SIGN_IN_API, gso)
.build();
gac.connect();
super.onStart();
}
}
Result Screen:
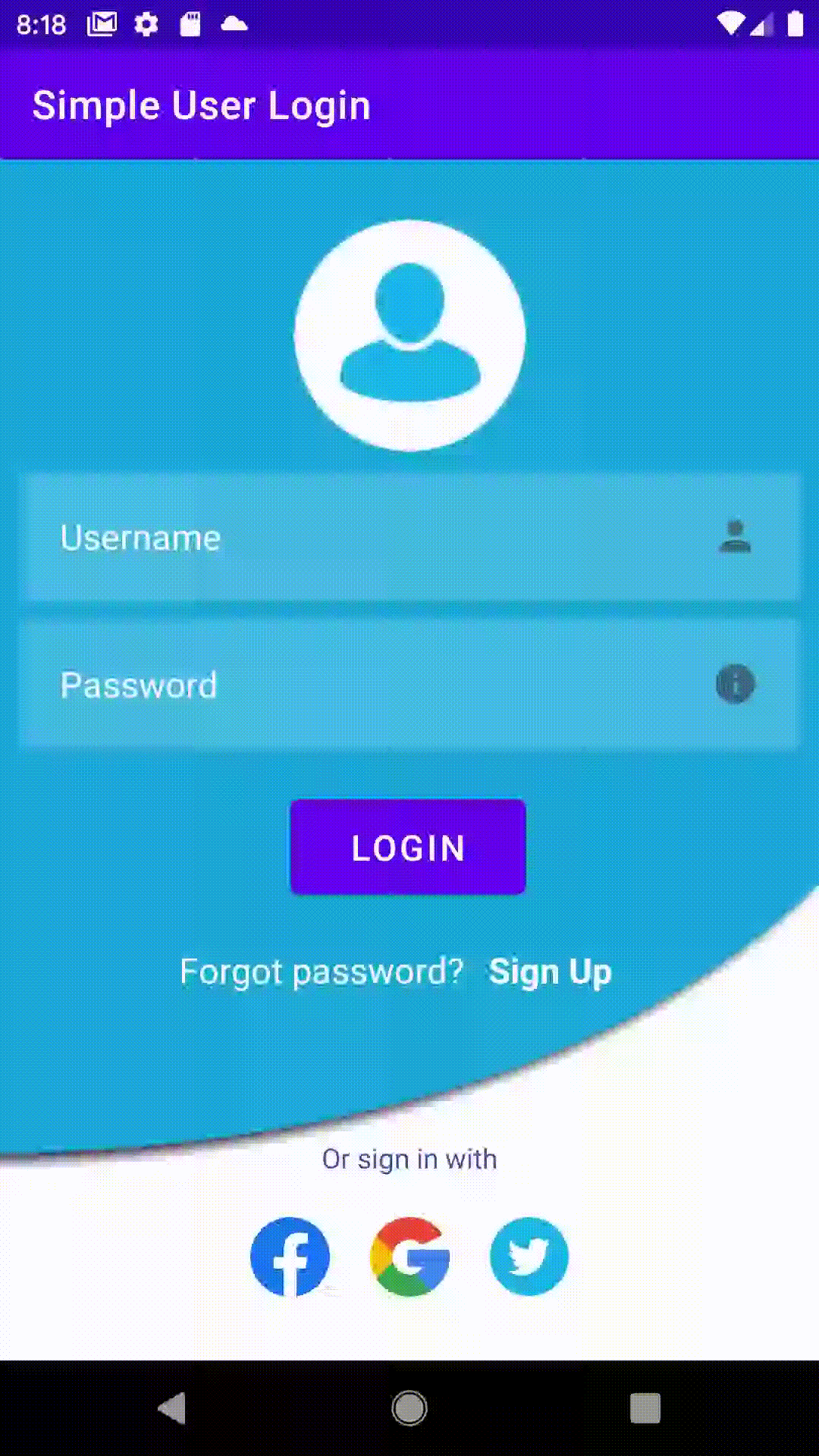