In HTML, You can customize Mouse Cursor with your image design, when you move on a page or object that you custom.
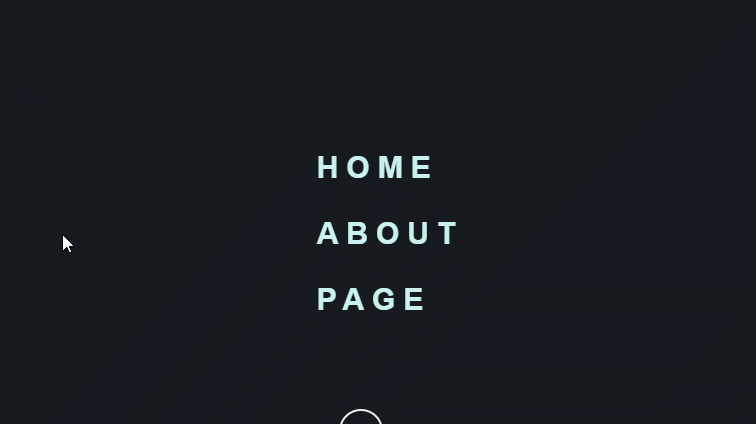
HTML Contents:
In this article, I will show you How to customize Mouse Cursor on a webpage using HTML, CSS, and JavaScript. In this article, I give credit to GTCoding. But I create HTML and add CSS (StyleSheet) with JS (Javascript) in a file, not split.
I create index.html with img folder, and in img folder has an image (custom-cursor.png), So you can download it.
Now, Please follow with me step by step:
1. Create index.html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
</head>
<body>
<div class="wrapper">
<nav>
<ul>
<li>
<a href="#">Home</a>
</li>
<li>
<a href="#">About</a>
</li>
<li>
<a href="#">Page</a>
</li>
</ul>
</nav>
<div class="cursor"></div>
</div>
</body>
</html>
2. Add CSS StyleSheet in Head tag <head></head>
<style>
* {
margin: 0;
padding: 0;
}
.wrapper {
background: #161a1d;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
nav ul {
list-style: none;
}
nav ul li {
margin: 32px 0;
animation: fade-down 2000ms forwards cubic-bezier(0.43, 0.53, 0.5, 1.02);
transform: translateY(-100px);
opacity: 0;
}
@keyframes fade-down {
30% {
opacity: 0;
}
100% {
opacity: 1;
transform: translateY(0);
}
}
nav ul li a {
color: #cbf3f0;
font-family: "Roboto", sans-serif;
font-size: 30px;
font-weight: bold;
text-decoration: none;
text-transform: uppercase;
letter-spacing: 8px;
position: relative;
}
nav ul li a::after {
content: "";
width: 0;
height: 8px;
background: #f5e502;
position: absolute;
bottom: -8px;
left: 0;
transition: all 500ms;
}
nav ul li a:hover::after {
width: 100%;
}
nav ul li a:hover {
cursor: url('img/custom-cursor.png'), auto;
}
.cursor {
position: fixed;
width: 40px;
height: 40px;
border-radius: 50%;
border: 2px solid #eee;
left: 0;
transform: translate(-50%, -50%);
pointer-events: none;
transition: width 1000ms, height 1000ms;
}
.cursor.large {
height: 70px;
width: 70px;
}
</style>
3. Add JavaScript in Body tag <body></body>
<script>
const cursor = document.querySelector(".cursor");
const links = document.querySelectorAll("nav ul li a");
const navlinks = document.querySelectorAll("nav ul li");
document.addEventListener("mousemove", (e) => {
let leftPosition = e.pageX + 4;
let topPosition = e.pageY + 4;
cursor.style.left = leftPosition + "px";
cursor.style.top = topPosition + "px";
})
links.forEach(link => {
link.addEventListener("mouseenter", () => {
cursor.classList.add("large");
})
})
links.forEach(link => {
link.addEventListener("mouseleave", () => {
cursor.classList.remove("large");
})
})
// Animation
navlinks.forEach((li, i) => {
li.style.animationDelay = 0 + i * 140 + "ms";
})
</script>
4. Completed Code HTML/CSS/JS
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Document</title>
<style>
* {
margin: 0;
padding: 0;
}
.wrapper {
background: #161a1d;
height: 100vh;
display: flex;
align-items: center;
justify-content: center;
}
nav ul {
list-style: none;
}
nav ul li {
margin: 32px 0;
animation: fade-down 2000ms forwards cubic-bezier(0.43, 0.53, 0.5, 1.02);
transform: translateY(-100px);
opacity: 0;
}
@keyframes fade-down {
30% {
opacity: 0;
}
100% {
opacity: 1;
transform: translateY(0);
}
}
nav ul li a {
color: #cbf3f0;
font-family: "Roboto", sans-serif;
font-size: 30px;
font-weight: bold;
text-decoration: none;
text-transform: uppercase;
letter-spacing: 8px;
position: relative;
}
nav ul li a::after {
content: "";
width: 0;
height: 8px;
background: #f5e502;
position: absolute;
bottom: -8px;
left: 0;
transition: all 500ms;
}
nav ul li a:hover::after {
width: 100%;
}
nav ul li a:hover {
cursor: url('img/custom-cursor.png'), auto;
}
.cursor {
position: fixed;
width: 40px;
height: 40px;
border-radius: 50%;
border: 2px solid #eee;
left: 0;
transform: translate(-50%, -50%);
pointer-events: none;
transition: width 1000ms, height 1000ms;
}
.cursor.large {
height: 70px;
width: 70px;
}
</style>
</head>
<body>
<div class="wrapper">
<nav>
<ul>
<li>
<a href="#">Home</a>
</li>
<li>
<a href="#">About</a>
</li>
<li>
<a href="#">Page</a>
</li>
</ul>
</nav>
<div class="cursor"></div>
</div>
<script>
const cursor = document.querySelector(".cursor");
const links = document.querySelectorAll("nav ul li a");
const navlinks = document.querySelectorAll("nav ul li");
document.addEventListener("mousemove", (e) => {
let leftPosition = e.pageX + 4;
let topPosition = e.pageY + 4;
cursor.style.left = leftPosition + "px";
cursor.style.top = topPosition + "px";
})
links.forEach(link => {
link.addEventListener("mouseenter", () => {
cursor.classList.add("large");
})
})
links.forEach(link => {
link.addEventListener("mouseleave", () => {
cursor.classList.remove("large");
})
})
// Animation
navlinks.forEach((li, i) => {
li.style.animationDelay = 0 + i * 140 + "ms";
})
</script>
</body>
</html>
Video tutorial:
Full source code, download here